Today’s Musical Selections
I hope you are all finding some "beauty in the broken American moment."
No? Try listening to some Hiss Golden Messenger.
Web APIs
Some examples for today are available here:
https://github.com/cs125-illinois/lecture-webapis-examples
Feel free to fork!
Review: Internet As Wired Infrastructure
The internet comprises an enormous amount of physical infrastructure.
-
Most of it is wired, not wireless. Wireless is mainly used for the first hop.
-
Most of it is fiber (glass), not copper. Signals degrade quickly in copper but travel faster and with less attenuation in glass 1. Copper is only used for the last 100 meters.
-
The internet wouldn’t exist without fiber optic cable. It’s one of the wonders of the modern world. It’s not just glass, it’s really clear glass.
Internet As Wireless Infrastructure
In recent years we’ve also built out a huge amount of wireless internet infrastructure.
-
Short range wireless is dominated by WiFi and what you use when you’re on campus, at home, or at a coffee shop
-
Medium-range wireless is used to provide connectivity to mobile devices like smartphones over longer distances. You usually buy this from a cellular provider like Verizon or Sprint.
Review: Internet As Connectivity
The result is that by connecting a computer to the internet, you are now connected to 4 billion other computers.
-
Many times the first connection is wireless
-
But after that point there is literally a wire that you can follow from your computer to the other computer
Review: The Internet Protocol (IP)
The Internet Protocol (IP) consists of a series of agreements that allow internet-connected devices to communicate.
-
What do we call each other? IP specifies the format of internet protocol addresses, also called IP addresses.
-
Here’s one:
192.17.96.8
(IPv4) -
Here’s a new one:
2607:f8b0:4009:807::2004
(IPv6)
-
-
How are our message structured? IP specifies a format for each message (or datagram) exchanged across the internet.

Packet Switching
Data is transferred over the internet in small units called packets.
-
Each packet may travel a different route between the source and destination
-
The internet protocol (IP) provides best effort packet transmission—but delivery is not guaranteed!
-
Internet routers are responsible for transferring packets one hop closer to their destination
-
Packet switching was revolutionary when it was proposed—but now even traditional voice traffic is moving to packet-based networks
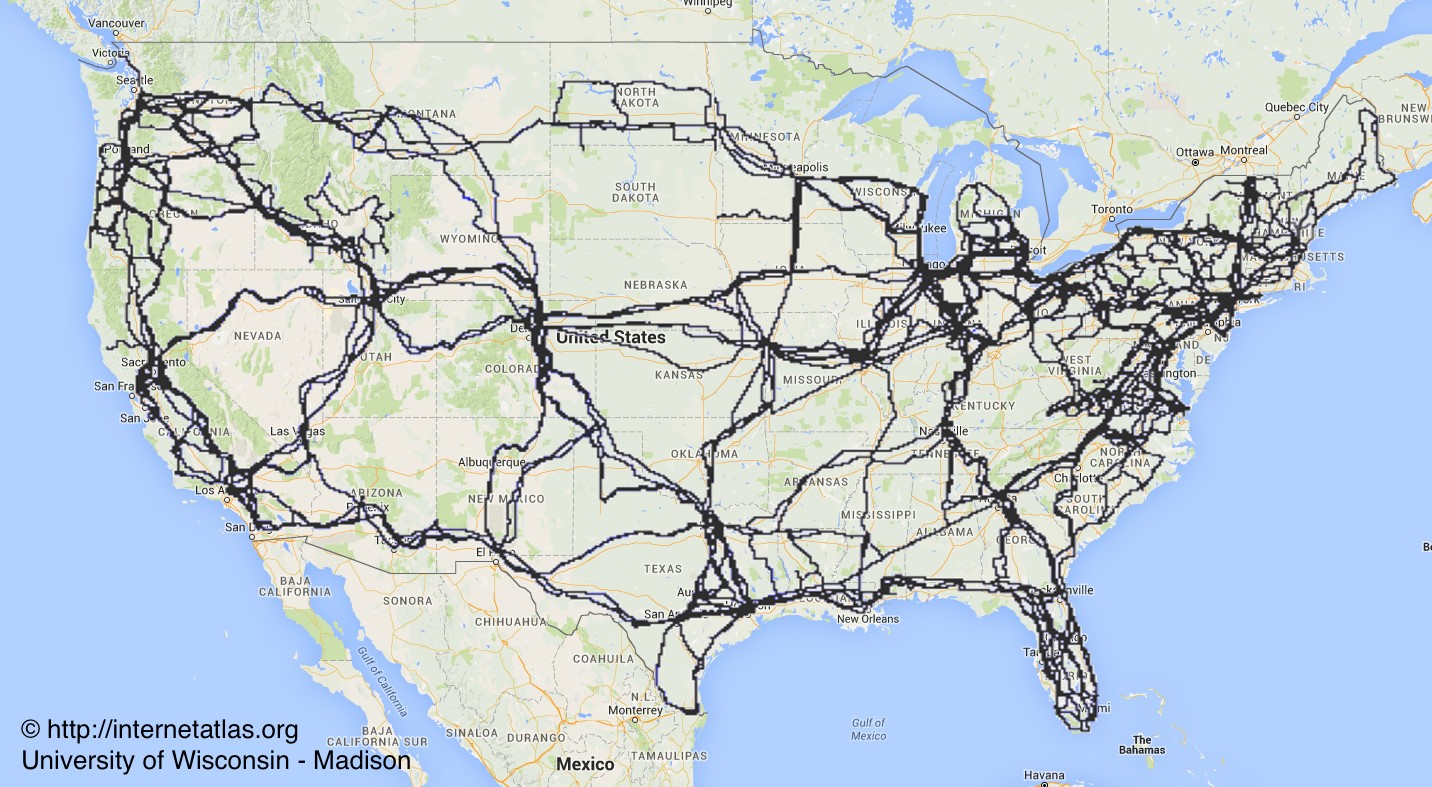
Internet Protocol Stack
So The Web Is Not The Internet
The web is just one of many services running over the internet.
What is the World Wide Web?
-
A protocol: the Hypertext Transfer Protocol (HTTP)
-
A markup language: the Hypertext Markup Language (HTML)
-
A styling language: Cascading Style Sheets (CSS)
-
A programming language: JavaScript
HTTP GET
and POST
HTTP defines many other types of requests, but GET
and POST
are by far the
most common.
-
Every time you load a web page it starts with a
GET
, and usually that’s followed by many otherGET
requests to fetch others parts of the page: style sheets, images, JavaScript code. -
Every time you submit a form it uses a
POST
to send data to the server, at which point your Facebook comment is recorded, or your credit card is charged and an package starts its way toward your house
HTTP: GET
v POST
The HTTP protocol specifies different semantics for GET
and POST
:
-
GET
should not change anything about the world, just return a document -
POST
should change something about the world—create a new account, pay your bill, purchase something, send a message, etc. -
As a result it is safe to repeat a
GET
but potentially problematic to repeat aPOST
: hence the "Do not click back" and "Do not submit this form twice" kind of warnings.
Web Page Contents: HTML
HTML defines how each page is structured:
<h1>This is a Simple Web Page</h1>
<p>
HTML includes both content and instructions to the browser determining
how the content should look. For example, the following items should be
in a numbered list:
</p>
<ol>
<li>First</li>
<li>Second</li>
<li>Third</li>
</ol>
<p>
<strong>Here is some bold text.</strong> <i>And this in italics.</i>
</p>
Web Evolution
The web has gone through many design changes over the years.
-
Static Sites: the web server returns a file from the disk that contains a complete web document
-
Example: most of
cs125.cs.illinois.edu
is a static website
-
-
Dynamic Sites: the web server runs code to produce an HTML document and respond to
POST
requests created by forms-
Example: sites like
my.cs.illinois.edu
are dynamic sites
-
-
Web Apps: most of the site is generated by JavaScript that runs in the user’s browser, with the server providing data as needed
-
Examples:
cs125.cs.illinois.edu/m/grades
, Discourse, Gmail, Google Docs
-
Web Page Contents: CSS
CSS defines how each page looks:
body {
font-family: sans-serif;
}
h1 {
font-size: 48px;
font-weight: bold;
}
Web Page Contents: JavaScript
JavaScript defines what each page does:
setInterval(function () {
var x = document.getElementById("title")
if (x.style.visibility === "visible") {
x.style.visibility = "hidden"
} else {
x.style.visibility = "visible"
}
}, 1000)
So What’s a Web API?
What’s An API?
In computer programming, an application programming interface (API) is a set of subroutine definitions, protocols, and tools for building application software.
In English, an API is a set of functions that perform a set of related and useful tasks.
Example API
Let’s say we wanted to find out the weather at a particular location:
// Get the current weather a particular location
static WeatherInfo getAtLocation(WeatherLocation location)
// Get the current weather a particular location and a particular time
static WeatherInfo getAtLocation(WeatherLocation location, Date date)
// Get a list of possible WeatherInfo objects for a given location string
static WeatherLocation[] searchLocations(String query)
Web APIs
A web API is just an API that you access over the web. Consider that:
-
We can send data to a web server using
POST
and also using URL parameters in aGET
request -
The web server can run code in response
-
And return a response, which does not have to be an HTML document
-
And in many cases custom internet protocols are blocked by firewalls, making it attractive to run APIs over HTTP
Web APIs: Sending Arguments
// Get the current weather a particular location
static WeatherInfo getAtLocation(WeatherLocation location)
To send the location
argument to the getAtLocation
function over the web we
have several options:
-
Stick it the URL:
/api/getAtLocation/(location)/
, which can be mapped to a function call -
Add it as a query parameter:
/api/getAtLocation?location=(location)
-
Use a
POST
request and put it in the body, possibly as JSON:
POST /api/getAtLocation/
{
"location": (location)
}
Web APIs: Returning Results
// Get the current weather a particular location
static WeatherInfo getAtLocation(WeatherLocation location)
In many cases web APIs return results using JSON (JavaScript Object Notation):
{
"consolidated_weather": [
{
"id": 6511056423747584,
"weather_state_name": "Thunder",
"weather_state_abbr": "t",
"wind_direction_compass": "E",
"created": "2018-04-09T02:37:19.655990Z",
"applicable_date": "2018-04-08",
"min_temp": -2.6099999999999999,
"max_temp": 2.2149999999999999,
"the_temp": 2.4950000000000001,
"wind_speed": 2.8707529204565336,
...
What’s Awesome…
Is that there are a gazillion public APIs out there. So go have fun!
What is REST?
You’ll often hear of REST or RESTful web APIs.
-
REST is a design pattern for creating web APIs.
-
URLs map to resources: so
GET
/products
returns a list of all products, whileGET
/products/10
get information about product with ID 10 -
HTTP verbs are meaningful:
GET
gets something,POST
creates a new entity,DELETE
removes one, etc. -
HTTP response codes are meaningful: 200 is
OK
, 405 is not authorized, etc. -
The bodies of requests and responses are in
JSON
REST Examples
Request | Meaning | Java-Like Function |
---|---|---|
|
Retrieve a list of all items |
|
|
Retrieve information about item 81 |
|
|
Retrieve a list of all items that are frisbees |
|
|
Create a new item |
|
More REST Examples
With two additional useful HTTP verbs: PUT
and DELETE
Request | Meaning | Java-Like Function |
---|---|---|
|
Update information about item 81 |
|
|
Delete item 81 |
|
Questions About Internet, Web, or Web APIs?
Internet Design Principles
The internet established many powerful and important design principles. One of the most important is the end-to-end principle:
In networks designed according to the end-to-end principle, application-specific features reside in the communicating end nodes of the network, rather than in intermediary nodes, such as gateways and routers, that exist to establish the network.
End-to-End Example: Reliable Delivery
Reliable delivery is not guaranteed by the core Internet Protocol.
-
Not every application needs it!
-
Moved to the endpoints: that is, implemented on your device and whatever computer you want to communicate with reliably.
End-to-End Principle: Consequences
The end-to-end principles has had powerful implications for internet design and evolution.
-
The core network stays simple
-
The core network doesn’t choose winners and losers
Net Neutrality
Net neutrality is essentially enshrining the end-to-end principle in law.
-
Internet service providers should not discriminate against traffic based on where it comes from, where it is going, or other features
-
This keeps the internet available for the kinds of transformative innovation it has supported since its creation.
This Will Be Your Problem Soon
Please do the right thing.
Questions about the Internet, Web, and Web APIs?
Announcements
-
This week’s quiz is on sorting, but the programming problems are not on sorting. Good luck!