More About Interfaces
> Click or hit Control-Enter to run Example.main above
Review: What Is An Interface?
Interface: a shared boundary across which two or more separate components of a computer system exchange information.
-
Interfaces can be between two pieces of software, between software and hardware, between computers and their users, or between various permutation of these components.
-
Interfaces enable different parts of a system to interact in a structured way.
Java Interfaces
public interface Add {
int add(int first, int second);
}
-
Java interfaces look like empty objects: just method signatures with no implementation.
-
Interfaces can declare both methods and variables.
-
However, interfaces variables are
public static final
by default, meaning that they are only useful for declaring constants.
Implementing Interfaces
public interface Add {
int add(int first, int second);
}
public class Adder implements Add {
public int add(int first, int second) {
return first + second;
}
}
-
Interfaces don’t do anything useful by themselves. Instead, they have to be implemented by specific classes.
-
To declare that a class implements an interface you use the
implements
keyword as shown above. -
To implement an interface you must implement all of the methods that it declares.
> Click or hit Control-Enter to run Example.main above
Interface Casting
public interface Add {
int add(int first, int second);
}
public class Adder implements Add {
public int add(int first, int second) {
return first + second;
}
public int multiply(int first, int second) {
return first * second;
}
}
Add add = new Adder();
System.out.println(add.add(10, 20));
// But this doesn't work because multiply is not part of the add interface
System.out.println(add.multiply(10, 20));
-
Similar to inheritance I can automatically cast an object reference to any interface that it implements.
-
However, if I do that I can no longer access methods that are not part of the interface.
> Click or hit Control-Enter to run Example.main above
Interfaces v. Inheritance
So far this seems very similar to inheritance and overloading.
-
The interface is like the parent class
-
implement
is likeextends
-
Providing your own implementation is like overriding a parent’s method
abstract
Methods
It’s actually even more similar than it seems.
Remember abstract
classes?
abstract
classes can also have abstract
methods:
public abstract class Add {
public abtract int add(int first, int second);
}
So Why Interfaces?
Added Flexibility
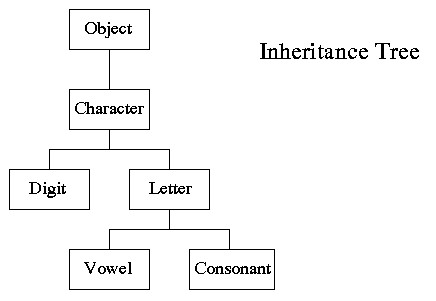
Sometimes we want to mix capabilities from different branches of the tree.
Multiple Inheritance
public interface Add {
int add(int first, int second);
}
public interface Subtract {
int subtract(int first, int second);
}
public class Mathy implements Add, Subtract {
public int add(int first, int second) {
return first + second;
}
public int subtract(int first, int second) {
return first - second;
}
}
Unlike inheritance, classes can implement multiple interfaces.
> Click or hit Control-Enter to run Example.main above
Interface as Contract
/**
* Compares this object with the specified object for order.
*
* Returns a negative integer, zero, or a positive integer as this object is
* less than, equal to, or greater than the specified object.
*/
public interface Comparable {
int compareTo(Object other);
}
Interfaces represent a contract between the interface provider and the interface user.
The interface represents all that the two components on either side need to agree on for things to work correctly.
Interface as Contract
public interface Comparable {
int compareTo(Object other);
}
By implementing
Comparable
you commit to being able to compare two instances of your class.
Using this ability I can implement code that:
-
sorts an array containing instances of your class
-
finds the maximum or minimum value of multiple instances of your class
-
arranges instances of your class into a binary tree 1
Interface as Abstraction Barrier
public interface Comparable {
int compareTo(Object other);
}
Good interfaces also represent a barrier between two unrelated parts of a computer program or system.
-
If I implement
Comparable
I don’t need to worry about how my implementation is used, but suddenly my class will have many new desirable features -
If I use
Comparable
I don’t need to worry about how the interface is implemented but I know that I can correctly compare two objects
Review: HW40
interface Comparable {
int compareTo(Object other);
}
Create a class called StringLength
that implements a version of the Java
Comparable
interface as shown above.
StringLength
wraps a String
and should provide a constructor that takes a
String
as a single parameter, which is stored internally by each instance of
StringLength
.
Review: Comparable
Comparable
allows objects to be put in order.
In this case, you should order StringLength
objects based on the length of
their String
instance variable.
Specifically compareTo
should return:
-
-1 if
other
is less than the specified object,null
, or not an instance ofStringLength
-
0 if
other
is equal to the specified object -
1 if
other
is greater than the specified object
(Note that this is backwards from the official Comparable
interface, but
the result is just a convention.)
> Click or hit Control-Enter to run Example.main above
It’s Good to Be Comparable
This small function is surprisingly powerful. It allows existing Java code to:
-
sort instances of your class
-
efficiently search for instances of your class
-
We’ll discuss both data structures and algorithms that utilize
Comparable
over the next few months
.equals
v. compareTo
In Java every object must provide .equals
, but only those that implement
Comparable
must provide .compareTo
.
Why?
-
Java decided that every class must have some notion of equality: at minimum, an object instance should be equal to itself!
-
In contrast, not every class supports meaningful comparisons
Comparable
or Not
What are some examples of classes that can or cannot be compared?
> Click or hit Control-Enter to run Example.main above
HW42
Create a class called Minimum
that provides a single class method min
.
min
should take an array of any object that implements Comparable
and return
the minimum value from the array, using compareTo
to compare object instances.
So the function signature of min
should be public static Comparable
min(Comparable[] values)
.
HW42
As a reminder, first.compareTo(second)
returns a positive value if first
is
larger than second
, a negative value if first
is smaller than second
, and
0 if they are equal.
Note that the array of Comparable
references passed to min
will all be the
same type and contain no null
references.
However, the array may be null
or empty in which case you should return
null
.
> Click or hit Control-Enter to run Example.main above
Announcements
-
We have a anonymous feedback form to the course website. Use it to give us feedback!