Introduction to Objects
> Click or hit Control-Enter to run the code above
Onward
These are the three main challenges that will occupy us for the remainder of the semester:
-
Algorithms: How do we use computers to solve problems?
-
Data structures: How do we structure information to enable efficient algorithms?
-
Software development: How do we write, debug, test, and publish good computer software?
How Do We Structure Good Computer Programs?
-
Break our code into reusable, testable, and understandable pieces
-
Combine state and behavior
-
Document our code appropriately
-
Reuse preexisting solutions as much as possible
-
Share our code with others!
Design
Programming is an intensely creative activity.
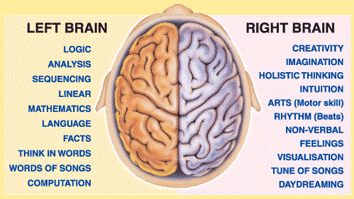
Design and Modeling
Java objects begin to expose you to the design aspects of computer science.
Ahead of us there are no right answers, just
-
creativity
-
imagination
-
holistic thinking
-
intuition
-
visualization
Objects
Objects combine state (like variables) and behavior (like functions).
-
What does it store? Objects allow us to structure data in more varied ways than we can achieve with primitive types alone.
-
What does it do? Objects allow us to place methods with the data that they naturally belong with.
Objects: Uniting Algorithms and Data
The two core concerns of computer science.
Object Definition
Object: In the class-based object-oriented programming paradigm, "object" refers to a particular instance of a class where the object can be a combination of variables, functions, and data structures.
Object Terminology: Class
class Person {
String name;
int age;
void printName() {
System.out.println(this.name);
}
}
A class determines how a particular type of object will behave.
You can think of the class as a blueprint that is used to create object instances of a particular type.
Object Terminology: Class
class Person {
String name;
int age;
void printName() {
System.out.println(this.name);
}
}
Instances of object class Person
:
-
Have a variable
name
of typeString
-
Have a variable
age
of typeint
-
Have a method
printName
that takes no arguments and returns nothing
Object Terminology: Class
class Person {
String name;
int age;
void printName() {
System.out.println(this.name);
}
}
Unlike other languages, Java classes cannot be modified after the program is compiled (at runtime).
-
This is frustrating when writing small programs
-
This is sometimes essential when developing large programs
Object Terminology: Instance
class Person {
String name;
int age;
void printName() {
System.out.println(this.name);
}
}
Person geoffrey = new Person();
An instance of a class is an object of that type. We create an instance using
the new
keyword.
-
Person
is a class—a type of object -
geoffrey
is an instance of typePerson
Dot Notation
class Person {
String name;
int age;
void printName() {
System.out.println(this.name);
}
}
Person geoffrey = new Person();
geoffrey.age = 38;
System.out.println(geoffrey.age);
We access an object’s state and methods using dot notation.
> Click or hit Control-Enter to run the code above
Instance Variables
class Dimensions {
int width; // I'm a primitive type
int height;
}
class Room {
String name; // I'm another object
Dimensions dimensions; // I'm defined above
}
Room diningRoom = new Room();
diningRoom.dimensions = new Dimensions();
diningRoom.dimensions.width = 10;
Instance variables can be both primitive types or other objects.
> Click or hit Control-Enter to run the code above
Objects as Data Structures
Sometimes known as records—but objects are much more than that…
Instance Methods
class Dimensions {
int width;
int height;
int area() {
return this.width * this.height;
}
}
Dimensions example = new Dimensions();
example.width = 10;
example.height = 20;
System.out.println(example.area());
Classes can also define methods that can be called on each instance.
> Click or hit Control-Enter to run the code above
this
class Dimensions {
int width;
int height;
int area() {
return this.width * this.height;
}
}
Instance methods can refer to their instance variables using the this
keyword.
this
refers to the instance that is executing the method.
> Click or hit Control-Enter to run the code above
Object Modeling
We frequently use Java objects to model real objects or entities.
Objects allow us to design software that deals with things in realistic and natural ways.
Midterm Preparation
Open-Ended Quiz Question
One question like this will appear on the midterm exam.
Announcements
-
All lecture videos and EMP sessions have been posted to YouTube—and we’re getting better at this, so lectures should be up quickly now
-
Next Monday will be midterm review, with the midterm exam starting Tuesday
-
Office hours for MP2 continue—please get it done, since it will help you prepare for the midterm exam next week
-
MP3 will come out next Friday and be due two weeks from the following Monday