Inheritance
> Click or hit Control-Enter to run Example.main above
Q4 Review: Type Casting
To force Java to convert a int
to an double
, you would…
Q4 Review: Constructor Syntax
class D {
double e;
double f;
D D(double setE) {
this.e = setE;
}
}
What describes a problem with the class D
as defined above?
Q4 Review: String Equality
class Car {
// Instance variables and constructors not shown
String d() {
return this.year + " " + this.make + " " + this.model;
}
boolean e(Car other) {
return this.make == other.make;
}
}
What is one potential problem with the code shown above?
Q4 Review: Construction and Execution
class TV {
public String brand;
private int width;
private int height;
}
class Example {
public static void main(String[] unused) {
TV huge = new TV();
huge.width = 10;
}
}
What will happen when Example.main
above is executed?
(Everyone got credit for this question…)
> Click or hit Control-Enter to run Example.main above
Inheritance
public class Pet {
protected String name;
protected String type;
public void printMe() {
System.out.println("I'm a " + this.type + " named " + this.name);
}
}
public class Dog extends Pet {
Dog(String name) {
this.name = name;
this.type = "Dog";
}
}
Dog chuchu = new Dog("Chuchu");
chuchu.printMe();
Java allows objects to inherit state and behavior from another class.
Inheritance Terminology
public class Pet { }
public class Dog extends Pet { }
public class Cat extends Pet { }
In Java we establish inheritance using the extends
keyword.
-
Dog
extendsPet
and so inherits state and behavior fromPet
-
Cat
also extendsPet
and so also inherits state and behavior fromPet
-
We sometimes call
Pet
Dog
's andCat
's parent class -
We sometimes call
Dog
andCat
Pet
's children
More Inheritance Terminology
public class Pet { }
public class Dog extends Pet { }
public class Mutt extends Dog { }
In Java we can have have multiple levels of inheritance.
-
Dog
extendsPet
and so inherits state and behavior fromPet
-
Mutt
extendsDog
and so inherits state and behavior fromDog
andPet
-
We sometimes call
Pet
andDog
Mutt
's ancestors -
We sometimes call
Dog
andMutt
Pet
's descendants
protected
public class Pet {
public String name; // Anyone can set me
private String secret; // Only I can set this value
protected String type; // My descendants can use this value
}
public class Dog extends Pet {
Dog(String name) {
this.name = name;
this.type = "Dog";
}
}
-
public
: the variable can be read or written by anyone -
private
: the variable can only read or written by methods defined on that class -
protected
: the variable can only read or written by methods defined on that class or its descendants
> Click or hit Control-Enter to run Example.main above
The Dirty Truth About protected
public class Pet {
protected String name;
}
public class Dog extends Pet {
Dog(String name) {
this.name = name;
}
}
public class Example {
public static void main(String[] unused) {
Dog chuchu = new Dog("Chuchu");
chuchu.name = "Xyz"; // This works...
}
}
-
protected
: the variable can read or written by methods defined on that class or its descendants… in any package -
protected
: the variable can also be read and written by any method in the same package
public
, private
, and protected
Variables:
-
public
: the variable can be read or written by anyone -
private
: the variable can only read or written by methods defined on that class -
protected
: the variable can be read or written by methods defined on any descendant of that class in any package or any class in the same package
Methods:
-
public
: the method can be called by anyone -
private
: the method can only be called by other methods on that class -
protected
: the method can be called by other methods defined on any descendant of that class in any package or any class in the same package
super
Constructor
public class Pet {
protected String type;
Pet(String setType) {
this.type = setType;
}
}
public class Dog extends Pet {
private String breed;
Dog(String setBreed) {
super("Dog");
this.breed = setBreed;
}
}
Java classes can access their parent’s constructor using the super
keyword.
This must be the first thing done in a child constructor.
> Click or hit Control-Enter to run Example.main above
Hierarchical Thinking
Why organize objects into a hierarchy?
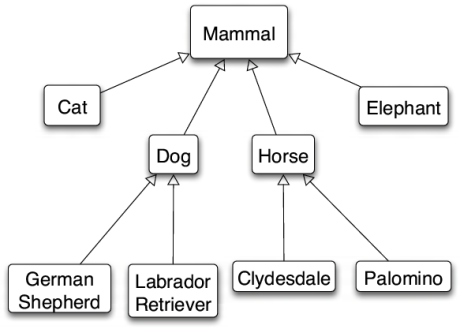
-
It can be a natural expression of real-world taxonomies
-
It allows us to organize and reuse code between multiple classes
The Tree Of (Java) Life
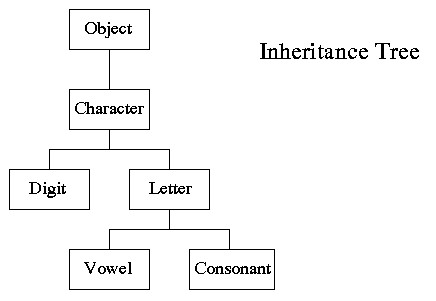
In Java, each class has a single parent, meaning that classes are organized into a tree.
If we follow each node to its parent, we eventually get to the top, or root…
The Root Object: Object
public class Dog { }
// is equivalent to
public class Dog extends Object { }
If a Java class
does not explicitly extend
another class, it implicitly
extends Object
.
Inherited from Object
public class Dog {
private String name;
Dog(String setName) {
this.name = setName;
}
}
public class Example {
public static void main(String[] unused) {
Dog chuchu = new Dog("Chuchu");
System.out.println(chuchu.toString());
}
}
All Java objects inherit a small number of important methods from Object
.
As a result, all Java objects implement these methods!
Methods Inherited from Object
For our purposes, the following methods inherited from Object
are important:
-
String toString()
: return aString
representing the instance. Frequently used for debugging. -
boolean equals(Object other)
: return aboolean
indicating whether this object is the same as another object -
int hashCode()
: return anint
uniquely representing an object’s contents. We’ll talk more about hashing later—it’s incredibly important and useful.
Method Overriding
public class Dog {
private String name;
Dog(String setName) {
this.name = setName;
}
public String toString() {
return this.name;
}
}
public class Example {
public static void main(String[] unused) {
Dog chuchu = new Dog("Chuchu");
System.out.println(chuchu.toString());
}
}
The default Object
methods are rarely useful.
So classes usually override them and provide their own.
Hierarchical Name and Method Resolution
The Java type hierarchy is used when resolving the names of variables and methods:
-
Does the class have a variable or method with the given name? If so, use it.
-
If not, search the parent class—but limited by
public
andprotected
-
Continue up the tree until the name is found or the search fails
> Click or hit Control-Enter to run Example.main above
Announcements
-
MP3 is due Friday.
-
My office hours continue today at 11AM in the lounge outside of Siebel 0226.