Strings
> Click or hit Control-Enter to run the code above
Q1 Review: Scope
int first = 5;
if (first > 4) {
int second = 4;
}
second++;
When the code above is finished executing, what is the value of second
?
Q1 Review: Forever
while (true) {
// loop contains code that is not shown here
}
True or false: the loop above will never terminate. Note that the code in the loop is not shown.
(Everyone got full credit for this question.)
Q1 Review: Scope Again
for (int index = 0; index < 10; index++) {
// do something
}
int count = index;
What is the value of count
when the code above finishes executing?
Q1 Review: continue
int counter = 0;
for (int index = 0; index < 10; index++) {
if (index > 4) {
continue;
counter--;
}
counter--;
}
What will the value of counter
be when the code above finishes executing?
Q1 Review: break
int counter = 0;
for (int index = 0; index < 10; index++) {
counter++;
if (index > 5) {
break;
}
}
What will the value of counter
be when the code above finishes executing?
Q1 Review: break
> Click or hit Control-Enter to run the code above
Storing Data
So far we know how to store a few different types of data using Java’s 8 primitive types:
-
Integers:
byte
,short
,int
,long
-
Floating point numbers:
float
,double
-
Character:
char
-
True or false:
boolean
What’s Missing?
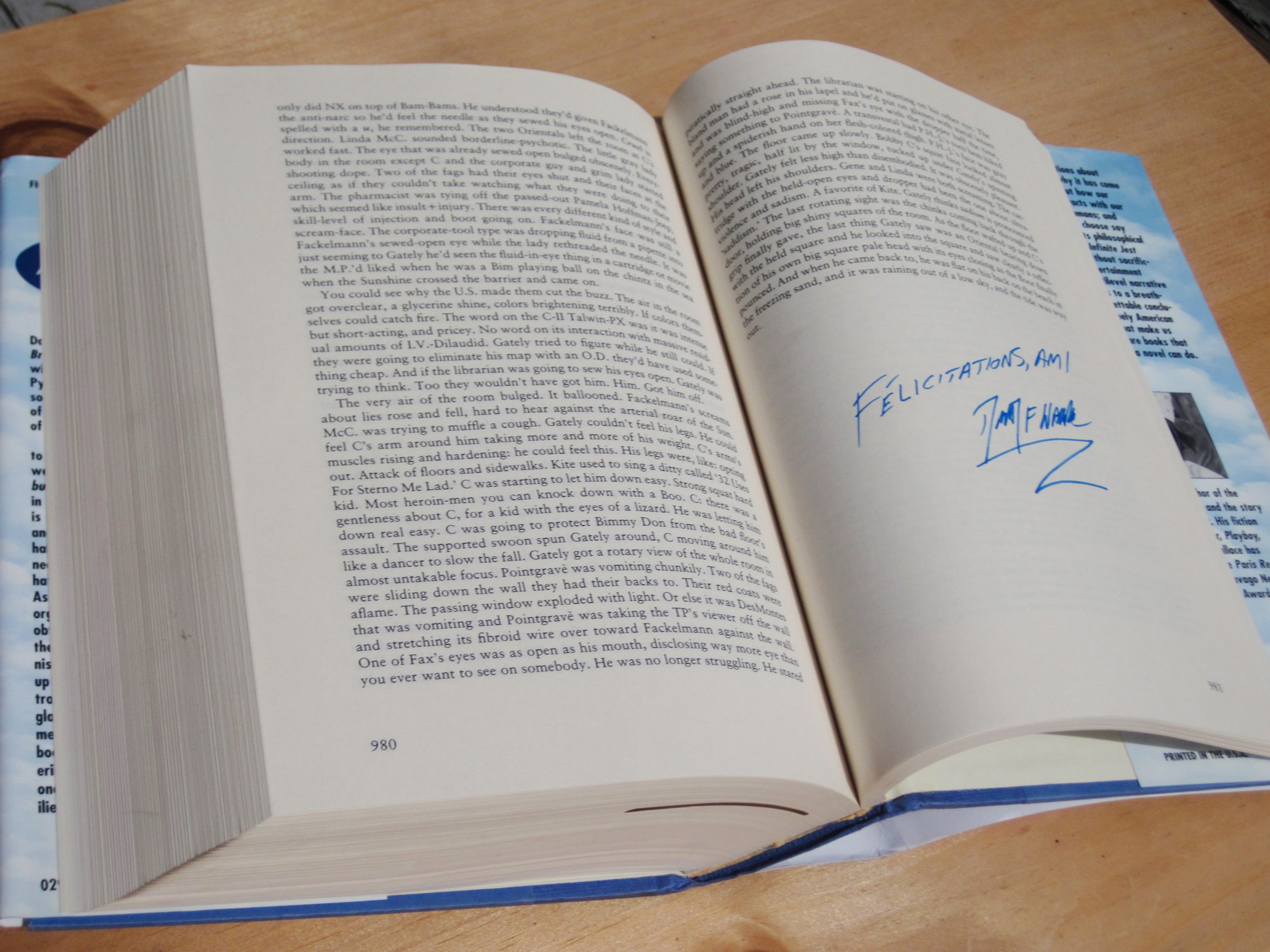


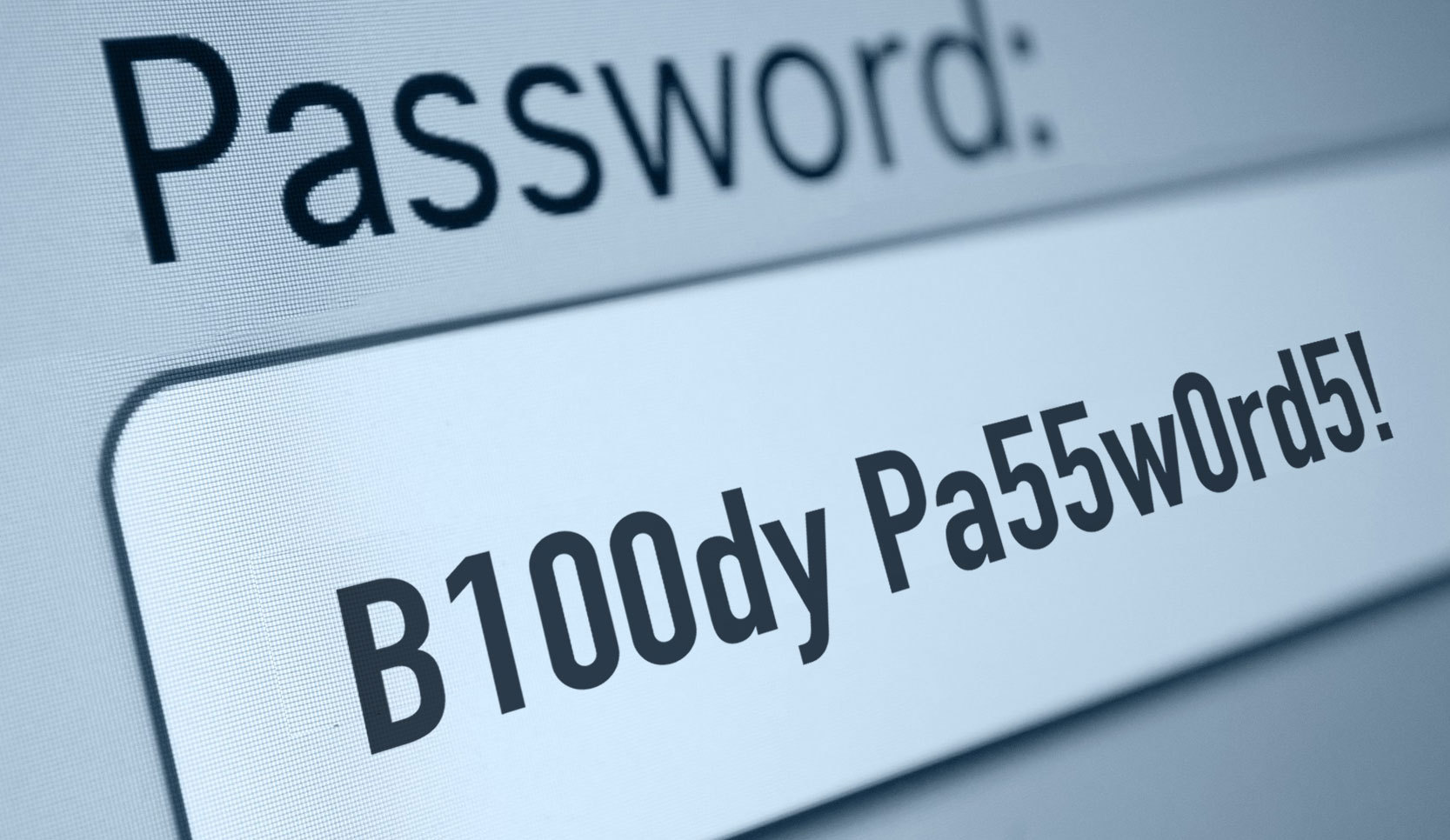
Strings
Much of the interesting data in our human world is in text form.
As a computer scientist, you call text a string.
(I realize that I cannot remember not knowing that term. I guess I’m old, or just have really become a computer scientist.)
Java Strings
Unsurprisingly, Java has a String
type.
We can declare, assign, and pass them to functions like primitive types.
String maybe;
maybe = "Challen"; // No, bad
maybe = "Geoff"; // Better, thanks
callMe(maybe); // You have my number?
Strings Seem Familiar, But Behave Differently
> Click or hit Control-Enter to run the code above
Our First Object
In Java a String
is our first example of an object.
Objects combine state (like variables) and behavior (like functions).
-
Each
String
has state: the array of characters in that string -
Each
String
also has behavior: functions that we can call on it that operate on its state
What does it store, and what does it do?
Objects v. Primitives
Primitives:
-
Store something that can be represented as a single number
-
Have type names that start with a lowercase letter:
int
,char
, etc.
Objects:
-
Can be made up of multiple other objects or primitive types
-
Have type names that start with an uppercase letter:
String
Objects
You will get a lot of practice working with and designing your own objects.
For now we’re going to use strings as a gentle introduction to using objects.
Strings
Normally to initialize an object in Java you use the new
keyword.
String myString = new String("ABC");
String anotherString = new String("DEF");
However, strings are so common that Java provides a shorthand:
String myString = "ABC";
String anotherString = "DEF";
(In practice there are minor differences between the two code snippets above, but we’re not going to worry about them.)
Arrays Are Also Objects
Do you remember? We’ve seen new
before…
int[] array = new int[8];
Java arrays are also objects—which is why we create them with new
and
can access their length property as array.length
.
Combining Strings
We can combine strings using the concatenation operator: +
.
String first = "Geoffrey";
String last = "Challen";
String full = first + " " + last;
Creating Strings
> Click or hit Control-Enter to run the code above
Dot Notation
We access an object’s state and methods using dot notation.
String example = "test";
System.out.println(example.length());
System.out.println(example.replace('t', 'b'));
System.out.println(example.toUpperCase());
Dot Notation
> Click or hit Control-Enter to run the code above
Fun With Strings
> Click or hit Control-Enter to run the code above
Questions About Strings?
Announcements
-
MP1 is out and due on Friday. Please get started!
-
Quiz scores are now available online.
-
The next set of Turing’s Craft exercises (TC4) are due tomorrow at midnight.