Primitive types, variables, and expressions
> Click or hit Control-Enter to run the code above
You’re Learning a New Language
And your computer is a very eager but unforgiving conversant
Our Approach
-
Start small: with just a few lines of code
-
Work up to slightly more complicated examples
-
Then to complete functions and algorithms
-
And eventually to understanding entire files of Java source code
What Are Computers Good At?
-
Basic math
-
Simple decision making
-
Doing things over and over again very, very fast
-
And storing data
Variable Declaration
// I declare a variable named c of type char
char c;
// I'm going to use an int and call it first
int first;
// I hereby create a boolean and shall name it isSet
boolean isSet;
-
In Java every variable has a name and a type
-
The name is for you, the programmer
-
The type is both for you and for Java
Variable Names Must Be Unique
// I declare a variable named c of type char
char c;
// This doesn't work. How will I tell them apart?
int c;
// Even this doesn't work if c has already been declared.
char c;
-
There are rules allowing the same name to be used in multiple places in the same program
-
We’ll learn about them later
Good Variable Names
Choosing good variable names will make your life a lot easier as a programmer.
Good variable names are:
-
Descriptive
-
Indicative of the variable’s function
-
As succinct as possible…
-
But see #2 above
-
This will make more sense as we go along
Comments
// Also: this is a single-line comment.
// It starts with a //
/*
* Here is another one. Multiline comments start with /* and end with
*/
// Comments are ignored by the computer, but can be
// some of the most important parts of your code
// They're for you and other humans
Variable Types
Java has eight primitive data types.
All other data in Java is represented by combinations of these building blocks. You can break them into four categories:
-
Integers:
byte
,short
,int
,long
-
Floating point numbers:
float
,double
-
Character:
char
-
True or false:
boolean
Variable Initialization
// I declare a variable named mine of type float
// and initialize it to 0.1
float mine = 0.1;
// Let there be a boolean called isItSnowing
// and initialize it to false
boolean isItSnowing = false;
// Declare timeSince1979 of type long
// and initially set it to 1204209
long timeSince1979 = 1204209;
Experimenting With Initialization
> Click or hit Control-Enter to run the code above
Literals
A literal is a number or other value that appears directly in the source code.
// 1000 is a long literal. Note the L suffix.
long big = 1000L;
// 'g' is a character literal.
char one = 'g';
// true and false are boolean literals.
boolean itsEarly = true;
boolean iSleptWell = false;
Variables Can Be Modified
> Click or hit Control-Enter to run the code above
Variables Must Maintain the Same Type
> Click or hit Control-Enter to run the code above
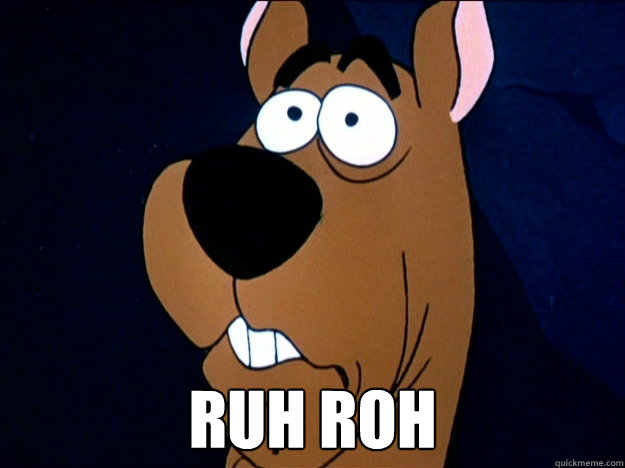
Variables Can Be Modified Using Other Variables
> Click or hit Control-Enter to run the code above
What Makes Primitive Types Primitive?
-
They can all be stored by the computer as a single number.
But wait… what about char
?

Our First Example of a Convention
There’s no law of the universe that says that the number 97 should represent 'a'.
It’s just what we’ve all agreed on.
We’ll discuss floating point representation in lab next week—it’s pretty cool.
Why Are There Multiple Numeric Types?
-
Integers:
byte
,short
,int
,long
-
Floating point numbers:
float
,double
Different types take up different amounts of computer memory and so can store different values.
Don’t worry too much about how things are stored yet. But the limits are important to understand.
Type Limitations
> Click or hit Control-Enter to run the code above
Why Types
-
Types force you to specify how much space you need to store your data. That can make your program more efficient
-
Types also help catch some common programming errors
Questions About Variables?
EMP
CS 199 EMP (Even More Practice) is a chance for you to get (even) more practice.
-
It’s held Monday nights from 5–7PM
-
You can register for it for one credit. If you do you’ll need to attend regularly.
-
You can also not register and show up when you want. It’s open to all.
-
More details on the website.
Announcements
-
Monday we will continue with the basics of imperative programming, including conditionals and loops
-
We are giving a quiz starting today in the CBTF covering course policies. Please sign up and take it.
-
MP0 is out and due a week from today! At least get your environment set up. Office hours until 5PM today.
-
Please fill out the intro survey! 1% extra credit for anyone who does by Monday 1/22 at noon.